Learning to code is scary!
Popular culture (especially films) makes you think you have to be a tech genius to understand coding.
It doesn’t help that code looks like gobbledygook! And it only gets worse with the incomprehensible jargon.
The good news is that you’re already good at the most difficult part of coding; Thinking!
To prove this, we’re going to write a program to count words.
You’ll realise that coding for primary school teachers doesn’t have to be scary!
Step 1 – Ensure you understand the problem
Even though I’m sure you already know how to count words. Let’s look at the problem anyway.
Read the sentence below and answer the question it poses.
How many words are in this sentence?
Your answer should be 7.
Can you explain how you identified each word? Have a think about it and then click to reveal some potential answers.
* I didn’t really think about it - I just counted the words.
* I recognised the words and counted them.
Those are both valid methods and you may have come up with a different one.
Now, take a look at the sentence below and count the number of words.
Τι λέει αυτό?
I’m guessing that you can’t read Greek. But I bet your answer is 3.
How did you count the words here? Especially if you can’t read Greek. Take a moment to think about it and then click to reveal my answer.
A space separates each word. So even though I don’t understand the words, I can see where each word begins and ends.
Step 2 – Find a general solution to the problem.
Let’s look at both sentences.
Is there a rule that we can come up with that will enable us to count the words in both sentences?
How many words are in this sentence? Τι λέει αυτό?
Think about it then click to reveal the answer.
You can count the spaces instead of the words.
So it would look like this.
How (1) many (2) words (3) are (4) in (5) this (6) sentence. (7) Τι (1) λέει (2) αυτό? (3)
Note: There is always one less space than words in a sentence. This can be fixed by adding an extra space at the end.
So now we have a rule that enables us to count words in both English and Greek. In fact it will work for dozens of languages including French, Italian, Spanish, Portuguese, and so on.
Step 3 – Turn your solution into an algorithm
Now let’s imagine we have to write some step-by-step instructions for someone to follow so they can count words in a sentence in any of these languages. What would the steps be?
You might describe it as follows: start at the first letter, and then check each letter in turn to see if it’s a space. Keep track of how many spaces we count.
Or in a step-by-step fashion:
1. set counter to 0 2. move to first letter of sentence 3. repeat until end of sentence 4. if letter is a space 5. add 1 to counter 6. move to next letter in sentence
Let’s see this in action.
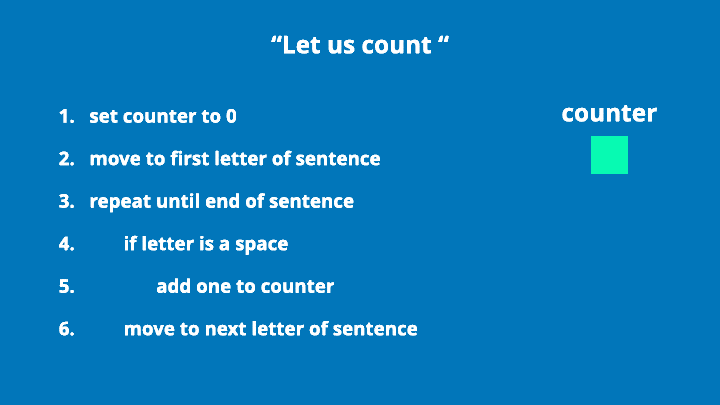
There is a formal definition for this list of steps. Do you know what it is?
Algorithm
A sequence of (step-by-step) instructions to complete a specific task, or solve a specific problem.
Yes, that’s right. The formal (or technical) name for those step-by-step instructions is an algorithm.
The classic example of an algorithm is a recipe. But wordless step-by-step instructions like Ikea, or Lego instructions are also algorithms.
Look at these activities that we do in our every day lives.
- Tying shoe laces
- Washing a sink full of dishes
- Adding multiple digit numbers
All these activities are algorithms. I bet you can think of multiple techniques for doing each of them. For example when adding multiple digit numbers you could use column addition, a calculator, or use one of many mental arithmetic strategies.
Now that we have an understanding of what Algorithms are lets investigate what coding (or programming) is.
Step 4 – Convert the algorithm into a program.
I’m guessing that when you think of people coding. You think of them typing instructions like those in the screenshot below into a computer.
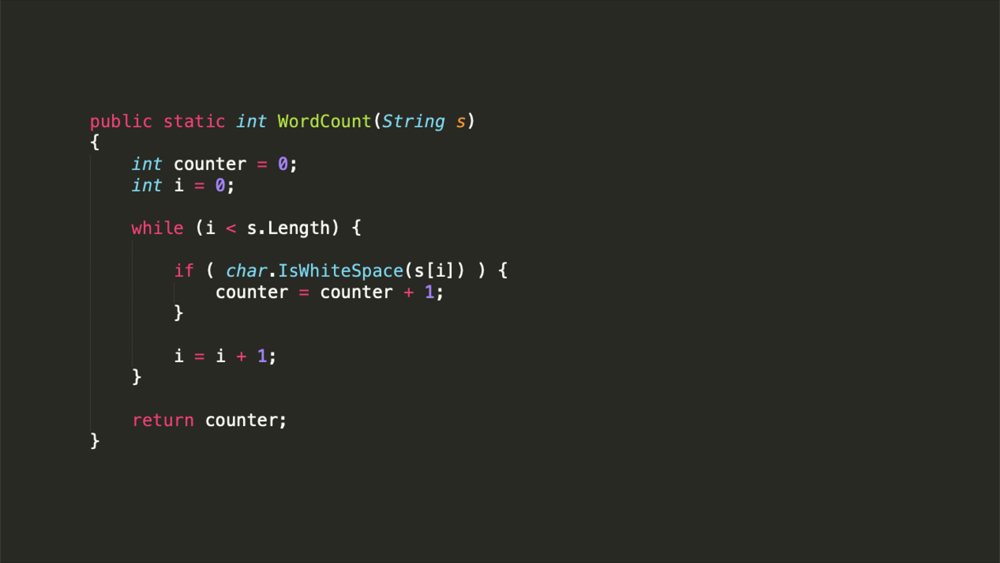
You may think that a major requirement is understanding what all the text means, and why it’s different colours. Or knowing why the lines are indented as they are. Or understanding what all the weird symbols (semi-colons, full-stops in odd places) mean.
And you’d be correct, but there’s more to it.
You could understand what all those things are, and still not know how to code.
So what is coding then?
It's problem solving.
To be more specific, it’s the whole process we just went through above from step 1 to step 3. Where we were trying to count the number of words in a sentence. This process ended with us being able to write step-by-step instructions (an algorithm) to explain how to do it.
Let’s review the process. We did the following:
- Manually counted the words in English.
- Counted the words in Greek (a language we can’t read).
- Looked for a rule that would work whilst counting words in both English and Greek.
- Identified that counting spaces was a simple substitute for counting words in both languages.
- Created step-by-step instructions to explain the process.
1. set counter to 0 2. move to first letter of sentence 3. repeat until end of sentence 4. if letter is a space 5. add 1 to counter 6. move to next letter in sentence
In other words we solved the problem of counting words in a sentence and came up with an algorithm to do so.
Notice that we did all this without using any coding terminology.
We could have done it all with pen and paper.
Coding often involves thinking through a problem in your head, on paper, or discussing with others. I solve my most tricky problems in the shower for example.
The final part, typing into a computer, is only done when you already know the solution (the algorithm).
Once we’ve done that, converting that algorithm into code is incredibly simple (if you understand the programming language).
Admittedly C# looks intimidating if you aren’t familiar with it and most people would think of this as the most difficult part.
Luckily, programming languages are much simpler to learn than real languages. They all follow simple rules, but once you’ve learnt the rules for one language it’s very easily transferable. They are all based on the same building blocks.
For example, I’ve used over 14 programming languages commercially and know even more. Yet I’m definitely not a “rockstar” coder. I have no innate talent for languages – I only know English.
Converting our algorithm into code
It’s outside the scope of this guide to teach you a programming language. So instead I’m going to show you how easy it is to convert a well written algorithm into code in two different languages.
Below is the same code that you saw above. I didn’t mention this before, but it’s actually the C# code of the algorithm that we created earlier in step 3.
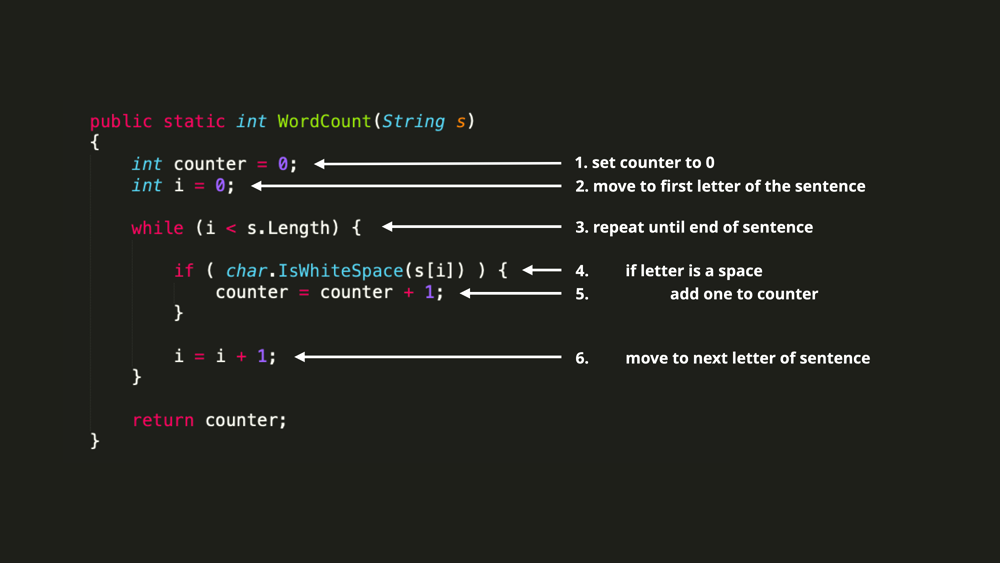
Notice how each step in our algorithm (on the right) corresponds to one line of code in the program.
I’m sure you’ll agree that if you understood the language it wouldn’t take much effort to convert the algorithm into a program. It’s simply a line for line substitution.
Let’s take a look at the code for another language that you are probably more familiar with. Scratch. It looks way less intimidating than C#. But because the example below is using the same algorithm, the program logic (the order of the commands) is exactly the same as the C# example above.
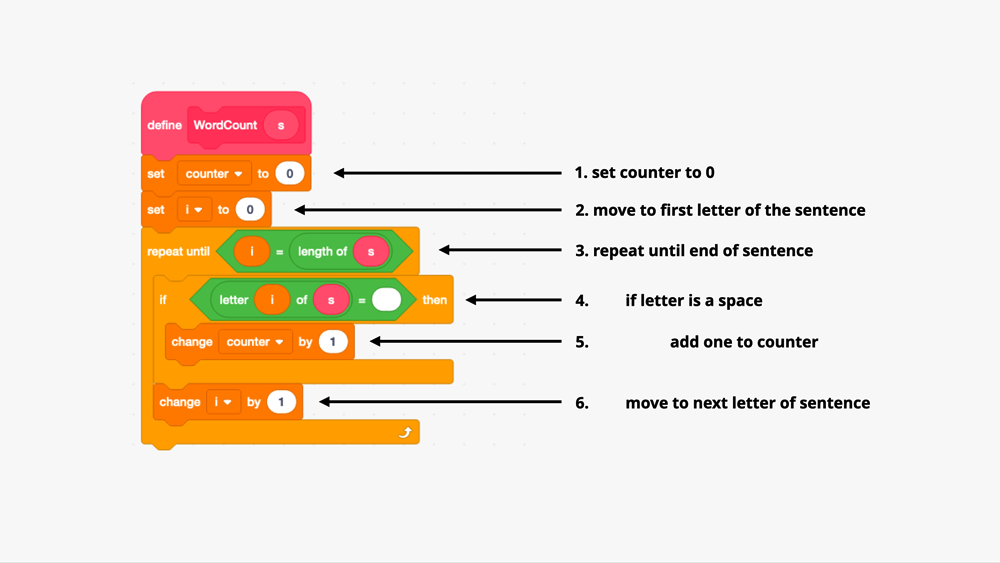
Notice again how each step in the algorithm corresponds to one line of code in Scratch.
What is a program?
We already defined an algorithm as…
Algorithm
A sequence of (step-by-step) instructions to complete a specific task, or solve a specific problem.
So do you know what the definition of a program is?
Program
A sequence of (step-by-step) instructions to make a computer (or robot) do something specific.
If you compare the two explanations you’ll notice that they are almost the same. The only difference is that a Program is created specifically to run on a computer.
Conclusion
The trick with coding is to always start with the problem, and make sure you understand it fully. The most important part of the coding process is everything you do before you start writing code. Writing code should always be the last thing you do.
Let’s review our 4 steps again:
Steps | What we did |
1. Make sure you understand the problem. | Counted the words in English and Greek manually. |
2. Try to find a general solution to the problem. | Figured out that counting spaces worked in both languages. |
3. Turn your solution into an algorithm. | Created the step-by-step instructions to count words. |
4. Convert the algorithm into a program. | Converted the algorithm into code (C# / Scratch). |
Some tips for your class:
- If they aren’t used to creating algorithms. Consider giving them ready-made algorithms and asking them to write programs based on them.
- Once they’re comfortable creating programs from algorithms. Encourage them to solve the problem by coming up with an algorithm before letting them test it on the computer.
1 thought on “Demystifying coding for primary school teachers in 4 easy steps”
This is really useful to discuss with staff and students in school. A great resource with answers to help. Love this platform designed by Chi.